Simple AWS Lambda Deployment with Terraform
Deployment and change management of AWS Lambda functions and their associated resources using an IaC tool like Terraform
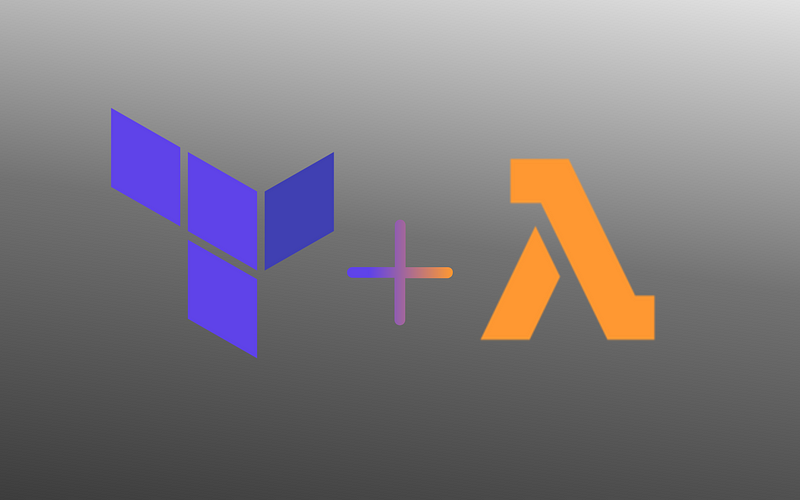
AWS Lambda is an Amazon Web Services (AWS) compute service offering that allows developers to write code in a variety of programming languages and deploy their code on a highly available and completely managed compute infrastructure.
AWS Lambda functions have become an essential component in many modern cloud applications and systems. Today, developers use AWS Lambda functions to service API requests for web and mobile applications, perform scheduled tasks, and bridge disparate applications and systems to list a few common uses.
It is therefore critical that good development and deployment practices be used with such a common system component. In another post, we shared 6 Tips for Working with AWS Lambda that highlight some of these best practices. One practice that was not discussed in that post was the deployment and change management of AWS Lambda functions and their associated resources using an infrastructure-as-code (IaC) tool like Terraform. This post provides an example of how to do this for a class of AWS Lambda functions I have taken to calling the simple lambda function.
A simple lambda function is one whose functionality is implemented primarily using the built-in modules for a particular programming language. Simple lambda functions may also use the Amazon SDK which is available in all AWS Lambda runtime environments. Furthermore, simple lambda functions are under 50 MB in size and can be zipped and directly uploaded to AWS Lambda.
The complete source code used in this post is available on GitHub.
The Python code below is for a simple lambda function that takes as input an event
object with a text
field containing a string value and returns a scrambled version of the input string in a JSON response.
Example
Input event
{ "text" : "rice and beans" }
Output (response)
{
"statusCode" : 200,
"headers" : { "Content-Type" : "application/json" },
"body" : "{\"result\": \"ircnnes a bdea\"}"
}
We begin by configuring the terraform provider for AWS. The code below assumes that your AWS credentials are available in the environment.
The following terraform code creates a zip archive of the simple lambda function’s source code and uploads it to the AWS Lambda service.
The file begins with a few lines declaring variables and initializing local identifiers that are used throughout. The last few lines of the file create an aws_iam_role
for the lambda function. But the core lines in this code are those for the aws_lambda_function
resource and the archive_file
data source.
The aws_lambda_function
resource is used to provision (as the name suggests) the AWS Lambda function. It utilizes the variables and local identifiers that were initialized and pulls information from the aws_iam_role
resource and archive_file
data source.
The archive_file
data source creates the zip archive of the simple lambda function’s source code located in the ./aws_lambda_functions/text_scrambler
directory. The directory structure below shows how the essential files in this example are organized.
.
├── aws.tf
├── aws_lambda_functions
│ └── text_scrambler
│ └── main.py
└── providers.tf
The configuration for the archive_file
data source to create the zip archive of the Lambda function’s source code is minimal in this example owing to the simplicity of the function’s code — i.e. the function only has one file in its source directory. However, the archive_file
data source is quite flexible and can accommodate archiving a more complex organization of code. For example, you can exclude certain files and set the octal file mode for all the archived files. Check out its documentation to learn more.
I encourage you to init
, plan
, and apply
the terraform code used in this post and play with deploying simple lambda functions using terraform.
terraform init
terraform plan -var="env_name=dev"
terraform apply -var="env_name=dev"
Summary
Infrastructure-as-code (IaC) tools such as terraform allow us to provision, manage, and change cloud infrastructure with much ease. AWS Lambda functions are a common component in many cloud-based applications and systems, and stand to gain all the benefits associated with IaC when managed using tools like terraform. This post demonstrated how to deploy a class of AWS Lambda functions I call the simple lambda function — i.e. AWS Lambda functions whose functionalities are implemented primarily using the built-in modules for a particular programming language and optionally the Amazon SDK.
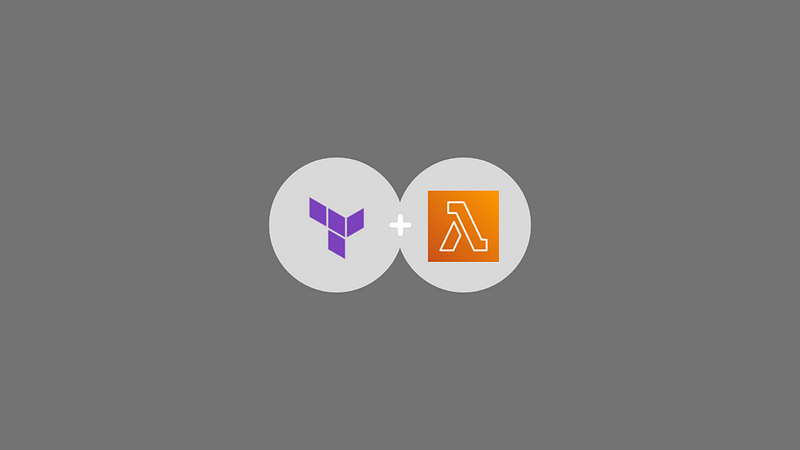
You can view the complete source code used in this post on GitHub.